@dan12345, here is what I’ve done.
I am using the Weather binding and pulling data from Yahoo. I also have a Webview with a compact summary of current conditions and the forecast. I’m using Yahoo’s condition icons in the sitemap. I also save temp and humidity values and present some charts in the sitemap.
I’m using Yahoo but the instructions purport to work with Wunderground, though the condition IDs might be different.
First I followed the instructions here to download and rename the weather condition icons for OH’s use and for making the map for the condition codes. However, these instructions have your item pull the data from Yahoo directly through the HTTP binding and applying an XSLT transform rather than getting the data through the Weather binding so I don’t use the transform. I use these icons for Condition_Id in my sitemap.
I am using rrd4j for persistence and charting, saving the charted items once a minute.
I can’t remember where I saw this example (maybe in the demo?) but I have some charts I use to see the weather, humidity, and pressure with a switch to move between three different charting periods (last hour, last day, last week).
Finally, I used the instructions at the bottom of the Weather Binding’s wiki page to create the webview of the current and next two days of the forecast. I customized it somewhat for my own purposes.
Here is my binding config:
# No API key when using Yahoo
weather:location.home.name=Home
weather:location.home.latitude=XX.XXXXXX
weather:location.home.longitude=XXX.XXXXXX
weather:location.home.provider=Yahoo
weather:location.home.language=en
weather:location.home.updateInterval=10
Items:
String Condition_Id "Weather is [MAP(yahoo_weather_code.map):%s]" { weather="locationId=home, type=condition, property=id" }
Number Weather_Temperature "Outside Temperature [%.0f] °F" <temperature> { weather="locationId=home, type=temperature, property=current, unit=fahrenheit" }
Number Weather_Humidity "Outside Humidity [%d%%]" <temperature> { weather="locationId=home, type=athmosphere, property=humidity" }
Number Weather_Pressure "Barometric Pressure [%.2f in]" <temperature> { weather="locationId=home, type=athmosphere, property=pressure, unit=inches" }
Number Weather_Pressure_Chart_Period "Chart Period"
Number Weather_Temp_Min "Today's Minimum [%.0f] °F" <temperature>
Number Weather_Temp_Max "Today's Maximum [%.0f] °F" <temperature>
Number Weather_Chart_Period "Chart Period"
DateTime Weather_LastUpdate "Last Update [%1$ta %1$tr]" <clock>
Number Weather_Humidity_Chart_Period "Chart Period"
DateTime Weather_Forecast_Day_1 "[%1$tA]" { weather="locationId=home, forecast=1, type=condition, property=observationTime" }
DateTime Weather_Forecast_Day_2 "[%1$tA]" { weather="locationId=home, forecast=2, type=condition, property=observationTime" }
Number Weather_Temp_Min_1 "Tomorrow's Minimum [%.0f] °F" <temperature> { weather="locationId=home, forecast=1, type=temperature, property=min, unit=fahrenheit" }
Number Weather_Temp_Max_1 "Tomorrow's Minimum [%.0f] °F" <temperature> { weather="locationId=home, forecast=1, type=temperature, property=max, unit=fahrenheit" }
Number Weather_Temp_Min_2 "Tomorrow's Minimum [%.0f] °F" <temperature> { weather="locationId=home, forecast=2, type=temperature, property=min, unit=fahrenheit" }
Number Weather_Temp_Max_2 "Tomorrow's Minimum [%.0f] °F" <temperature> { weather="locationId=home, forecast=2, type=temperature, property=max, unit=fahrenheit" }
Rules:
import org.openhab.core.library.types.*
rule "Update max and min temperatures"
when
Item Weather_Temperature changed or
Time cron "1 0 0 * * ?" or
System started
then
val startOfDay = now.toDateMidnight
if(Weather_Temperature != null) {
postUpdate(Weather_Temp_Max, Weather_Temperature.maximumSince(startOfDay).state)
postUpdate(Weather_Temp_Min, Weather_Temperature.minimumSince(startOfDay).state)
}
end
rule "Records last weather update time"
when
Item Weather_Temperature received update
then
postUpdate(Weather_LastUpdate, new DateTimeType())
end
Sitemap:
Frame label="Weather" {
Text item=Condition_Id icon="yahoo_weather" {
Frame {
Text item=Weather_LastUpdate visibility=[Weather_LastUpdate>30] valuecolor=[Weather_LastUpdate>120="orange", Weather_LastUpdate>300="red"]
Text item=Weather_Temperature valuecolor=[Weather_LastUpdate=="Uninitialized"="lightgray",Weather_LastUpdate>90="lightgray",>77="orange",>60="green",>40="aqua",<=40="blue"]{
Frame {
Text item=Weather_Temp_Max valuecolor=[>77="orange",>60="green",>40="aqua",<=40="blue"]
Text item=Weather_Temp_Min valuecolor=[>77="orange",>60="green",>40="aqua]",<=40="blue"]
}
Frame {
Switch item=Weather_Chart_Period label="Period" mappings=[0="Hour", 1="Day", 2="Week"]
Image url="http://localhost:8080/rrdchart.png?items=Weather_Temperature,Weather_Temp_Max,Weather_Temp_Min&period=h" refresh=6000 visibility=[Weather_Chart_Period==0, Weather_Chart_Period=="Uninitialized"]
Image url="http://localhost:8080/rrdchart.png?items=Weather_Temperature,Weather_Temp_Max,Weather_Temp_Min&period=D" refresh=30000 visibility=[Weather_Chart_Period==1]
Image url="http://localhost:8080/rrdchart.png?items=Weather_Temperature,Weather_Temp_Max,Weather_Temp_Min&period=W" refresh=30000 visibility=[Weather_Chart_Period==2]
}
}
Text item=Weather_Humidity {
Frame {
Switch item=Weather_Humidity_Chart_Period label="Period" mappings=[0="Hour", 1="Day", 2="Week"]
Image url="http://localhost:8080/rrdchart.png?items=Weather_Humidity&period=h" refresh=6000 visibility=[Weather_Humidity_Chart_Period==0, Weather_Humidity_Chart_Period=="Uninitialized"]
Image url="http://localhost:8080/rrdchart.png?items=Weather_Humidity&period=D" refresh=30000 visibility=[Weather_Humidity_Chart_Period==1]
Image url="http://localhost:8080/rrdchart.png?items=Weather_Humidity&period=W" refresh=30000 visibility=[Weather_Humidity_Chart_Period==2]
}
}
Text item=Weather_Pressure {
Frame {
Switch item=Weather_Pressure_Chart_Period label="Period" mappings=[0="Hour", 1="Day", 2="Week"]
Image url="http://localhost:8080/rrdchart.png?items=Weather_Pressure&period=h" refresh=6000 visibility=[Weather_Pressure_Chart_Period==0, Weather_Pressure_Chart_Period=="Uninitialized"]
Image url="http://localhost:8080/rrdchart.png?items=Weather_Pressure&period=D" refresh=30000 visibility=[Weather_Pressure_Chart_Period==1]
Image url="http://localhost:8080/rrdchart.png?items=Weather_Pressure&period=W" refresh=30000 visibility=[Weather_Pressure_Chart_Period==2]
}
}
Webview url="/weather?locationId=home&layout=example&iconset=colorful" height=7
}
}
Webview HTML:
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-type" CONTENT="text/html; charset=utf-8">
<link rel="stylesheet" type="text/css" href="weather-data/layouts/example.css" />
<script type="text/javascript" src="weather-data/layouts/example.js"></script>
</head>
<body id="weather-body" onload="formatIframe()">
<div id="weather-location-name">${config:name}, ${item:Weather_LastUpdate} Sunrise: ${item:Sunrise_Time} / Sunset: ${item:Sunset_Time}</div>
<table id="weather-table">
<tr>
<td rowspan="2"><img id="weather-icon" src="weather-data/images/${param:iconset}/${weather:condition.commonId}.png"/></td>
<td id="weather-temp">${item:Weather_Temperature.state}</td>
<td id="weather-temp-sign">°F</td>
</tr>
<tr>
<td colspan="2">
<table id="weather-table-details">
<tr>
<td>Humidity:</td>
<td>${weather:atmosphere.humidity}%</td>
</tr>
<tr>
<td>Pressure:</td>
<td>${item:Weather_Pressure}</td>
</tr>
</table>
</td>
</tr>
</table>
<table id="weather-forecast-table">
<tr>
<td>Today</td>
<td>${item:Weather_Forecast_Day_1}</td>
<td>${item:Weather_Forecast_Day_2}</td>
</tr>
<tr>
<td><img src="weather-data/images/${param:iconset}/${forecast(0):condition.commonId}.png"/></td>
<td><img src="weather-data/images/${param:iconset}/${forecast(1):condition.commonId}.png"/></td>
<td><img src="weather-data/images/${param:iconset}/${forecast(2):condition.commonId}.png"/></td>
</tr>
<tr>
<td class="temp-max">${item:Weather_Temp_Min} °F</td>
<td class="temp-max">${item:Weather_Temp_Min_1} °F</td>
<td class="temp-max">${item:Weather_Temp_Min_2} °F</td>
</tr>
<tr>
<td class="temp-min">${item:Weather_Temp_Max} °F</td>
<td class="temp-min">${item:Weather_Temp_Max_1} °F</td>
<td class="temp-min">${item:Weather_Temp_Max_2} °F</td>
</tr>
</table>
</body>
</html>
What it looks like:
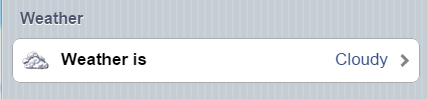
etc.
Hope this helps.
Rich
EDIT: Fixed an error in the openhab.cfg section. I needed to have:
weather.location.home.provider=Yahoo
weather.location.home.language=en
not
weather.location.provider=Yahoo
weather.location.language=en